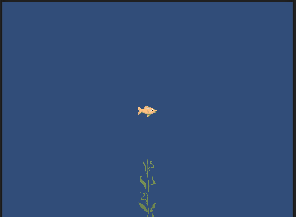
Unity3D – Physics & Collisions
In this part, we’ll add some seaweed and learn about the Unity3D physics system. We’ll learn how to handle collisions in Unity3D and kill some fish.
Let’s get started
Now that we have a fish that jumps in place, let’s add something for him to jump over. You may have noticed in the Art folder we have a sprite named “seaweed”. Let’s place a seaweed in our scene by dragging it from the Art folder (in the Project View) over to the Scene View.
Once it’s placed, let’s adjust the position in the inspector. We’ll set the X to 0 and the Y to -6.
Let’s Play
Before you hit play, think for a second about what you expect to happen.
- Will the fish fall through the seaweed?
- Will he hit it and die?
- Will he land on top?
- Once you’ve picked an answer, hit play and watch what actually happens.
What’s Missing?
If you guessed that the fish would fall through, great job. If not, let me explain why it happened. For our fish to collide with the seaweed, we need to tell the Unity3D physics engine that these GameObjects should collide. Luckily, that’s a very simple task for us to accomplish. What we need to do first is add a collider to our fish. A collider is just another component and we add it just like any other component.
- Select the Fish.
- In the inspector, hit Add Component.
- Find the PolygonCollider2D and add it.
Once you add the Collider, your fish should get a green outline.
Let’s think again. If we hit play now, what will happen? If you’re not sure, give it a try.
The fish is still falling through. You probably already guessed, but what’s missing is a collider on the seaweed.
Let’s add one now.
The Seaweed
Select the seaweed, then add a Polygon Collider2D, just like we did with the fish earlier.
You should notice the collider on the seaweed.
Now hit play one more time. I promise something will happen now.
If you want to see why the fish is rolling off the side, separate out your scene and game view.
To separate the views, just left click and hold the mouse button. While you’re holding the button, drag the mouse and the panel will detach. If you move to the right a bit, you should be able to dock them side by side.
Now hit play again and watch the fish falling along the collider of the seaweed.
Collision Handling
What we need to do now is handle the collision. The physics engine in Unity3D can detect collisions or make things roll and bounce on it’s own, but what we want to do is make the player lose the game. In Flappy Bird, if your bird touches a tube, you lose the game. We want to do the same thing here. To do that, we need to create a new script and attach it to our seaweed.
Create a new C# script in the Code folder.
Name it “SeaweedCollisionHandler”
Double click the “SeaweedCollisionHandler” file to open it in our editor (MonoDevelop or Visual Studio).
The file should look like this.
using UnityEngine; using System.Collections; public class SeaweedCollisionHandler : MonoBehaviour { // Use this for initialization void Start() { } // Update is called once per frame void Update() { } }
Since we won’t be using Start & Update on the SeaweedCollisionHandler, let’s delete them and add in some collision handling code instead.
Change your file to match this.
using UnityEngine; using System.Collections; public class SeaweedCollisionHandler : MonoBehaviour { void OnTriggerEnter2D(Collider2D other) { Debug.Log("Entered"); } }
Save the file then go back to the Unity3D Editor.
Select the “seaweed” GameObject in the Heirarchy then add the “SeaweedCollisionHandler” script to it in the inspector.
Next, on the PolygonCollider2D component, check the IsTrigger checkbox.

Seaweed with SeaweedCollisionHandler Script
Now hit Play and watch what happens.
Because we checked IsTrigger, the fish no-longer rolls off the seaweed.
Instead, it calls into our OnTriggerEnter code that we added to the SeaweedCollisionHandler.
And in our OnTriggerEnter code, we write a line of text to our debug console.
To view the debug console, just click the Console tab next to the Project tab.
You should see a single line of text in there that says “Entered”.
If you didn’t get that, double check that IsTrigger is checked and that the SeaweedCollisionHandler is attached to the correct GameObject (“seaweed”).
Now let’s Save our Scene
Before we save, let’s create a folder for Scenes
To create the folder, just right click and select Create->Folder
Now go to the File menu and select Save Scene As
Browse to the Scenes folder and Name our Scene “Fish“, then hit Save.
Time to Die
We really want our fish to die when he hits the seaweed (at least we do if we want our game to be like flappy bird).
Let’s go into the SeaweedCollisionHandler script and make it happen!
Edit the script to look like this:
using UnityEngine; using System.Collections; using UnityEngine.SceneManagement; public class SeaweedCollisionHandler : MonoBehaviour { void OnTriggerEnter2D(Collider2D other) { SceneManager.LoadScene(0); } }
That’s it. Now play the game and watch what happens.
When you hit the seaweed, your fish appears in the starting location again.
This happens because we’re reloading our level. (level & scene are interchangable terms for the Unity engine)
The LoadLevel command will load a Scene by Name or Index.
In this instance, we’re using the Index 0. (that just means it’s the first in the list of our scenes. 1 would be the second scene, 2 would be the third)
Application.LoadLevel(0);
Because we don’t have our project setup with a list of scenes, it’s just using our current scene.
Let’s change that now
Under the File Menu, select Build Settings…
Click the Add Current button.
You should see your scene added to the list.
Saving our Project
Up to this point, we haven’t really done anything that required us to save our project. We’ve saved our scene and scripts, but the project itself hasn’t been saved since we created it.
Because we changed some project settings, we need to save the project to make sure those settings aren’t lost.
When you were setting up your build options, you may have already seen the Save Project button.
Go ahead and hit that now to save our new settings.
Click File, then Save Project.
A saving dialog will pop up and disappear shortly after. If your computer is fast, you may not even notice it, but your project should be saved now.
Great work so far. In the next part, we’ll turn this into an actual game. We’ll add more seaweed, and start making the fish swim through them.