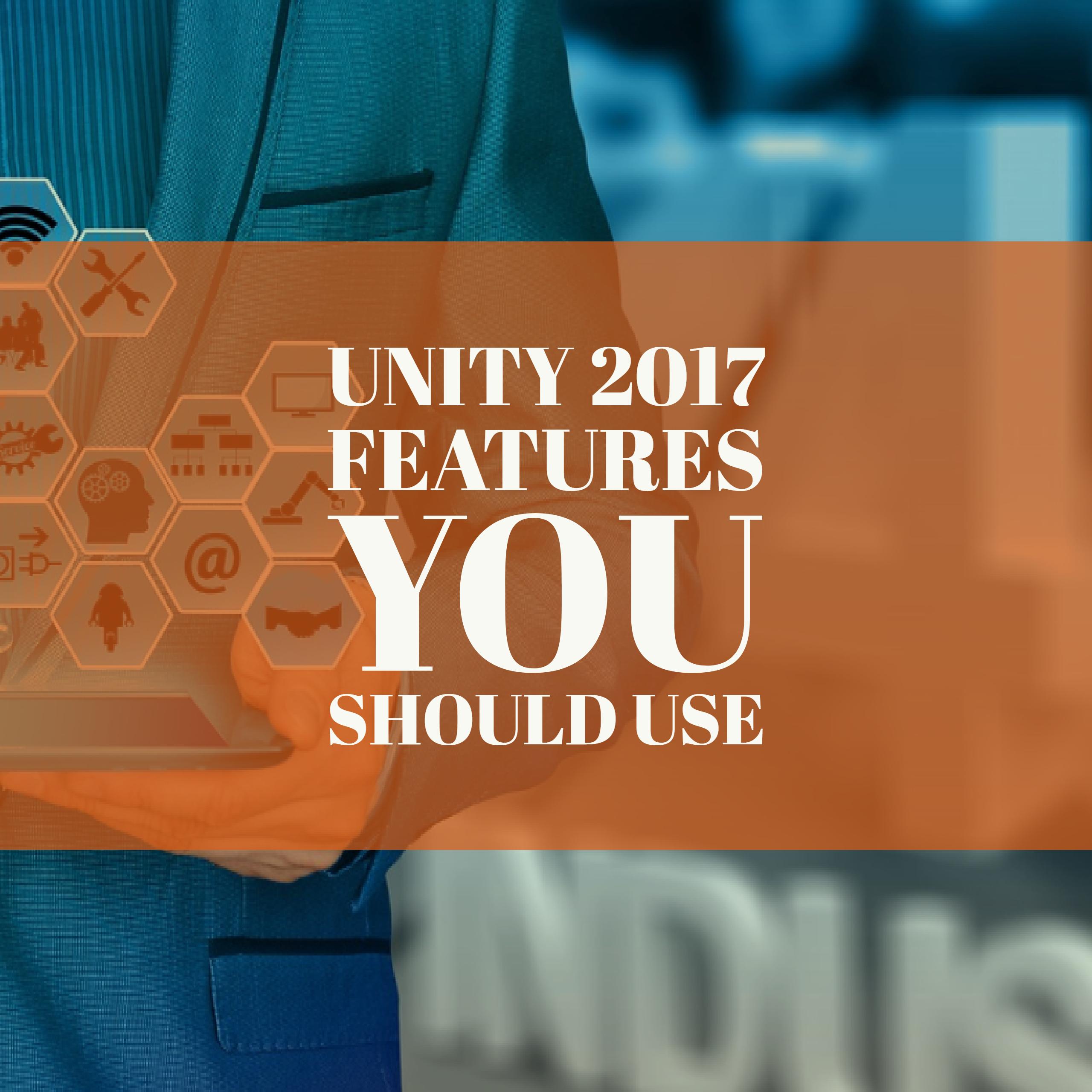
With the release of Unity 2017.1, the .net framework update to 4.6 has been included and is now just about ready for mainstream usage. The .net framework upgrade is actually pretty big, it contains a lot of things under the hood, and quite a few new pieces of functionality you can use. In this post, I’m only going to cover two of them, they’re two of my favorite. That’s because they’re extremely easy to start using right away and will make your code just a tad bit cleaner by removing some of the extra ceremony that was required before them. These features are String Interpolation (using the $ operator) and the Null Conditional Operator (aka Elvis operator ?. )
Important Warning
Before you upgrade to .net 4.6, be sure to commit / backup your project. The last time I did it, I ended up with quite a few prefab references becoming null. I don’t know if that’s a fixed issue, was a 1 time occurrence, or something else, but treat it just like you would a major version upgrade in Unity. Commit to source control and save yourself from possible headaches.
To demonstrate these, I plucked a line of code from one of my real projects that happens to use them both, for a very common use.. logging.
Before I show them off though, I want you to take a quick look at this
Here, I’m writing to the logs when the character handling the puck has changed (this is a hockey game). I log out the character that’s handling the puck change at {0} and the character that now is holding the puck with {1}. Sometimes the puck is held by nobody though, so I need to do a null check and pass in an empty string if that’s the case.. so I’m using the ternary / ? operator. In all, it’s 3 lines (sure it could be one long one).. and if you’re reading it for the first time, it takes a second.. or a few.. to look at, see what’s going on, make sure that ternary is correct.
Now let’s compare that to the .net 4.6 version.
The first thing to note is we no-longer have string.Format, but instead we start the string with a $ before it. This tells the compiler that we’ll be putting variables in our string using the { }’s. In-fact, right after the quote, we start with the first parameter.. and instead of a {0} that we have to look to the end for, we can put the variable right there. Behind the scenes, the compiler will treat this just like a string.Format from the first version, but we don’t have to mentally track the {0} to the first parameter and so on.
We do the same for the second parameter, but there’s a little something extra. In the 2nd parameter, we needed to check to make sure the heldBy wasn’t null. With the Null Conditional Operator, we can tell the compiler to do that for us. If the heldBy variable is null, it’ll be a null string. If it’s not, it’ll evaluate the gameObject and then the .name of that gameObject. All without us writing extra null checks.
It’s also worth noting that the Null Conditional Operator works for executing methods… or invoking events. You could have a method named Shoot() on a character, and call it with character?.Shoot() to have the code shoot if a character is assigned to the variable.. Not the best example, but hopefully you get the idea 🙂
Video Version